What is Sorting ?
Sorting is any process of arranging or in a sequence ordered of items list in a certain order. Like Numerically or Alphabetically order.
Sorting Function
- sort
- rsort
- asort
- arsort
- ksort
- krsort
1. Sort sort(array)
This function sorts an array. Elements will be arranged in Ascending order from lowest to highest when this function has completed.
Example Code:
//Without Sorting $colors = array(" White", " Black", " Grey", " Yellow", " Red", " Blue", " Green", " Brown", " Pink", " Orange", " Purple"); echo "<p>"; foreach ($colors as $key => $value) { echo($key == 0) ? $value : ",".$value; } echo "</p>"; //White, Black, Grey, Yellow, Red, Blue, Green, Brown, Pink, Orange,Purple //After Sorting Array in Ascending Alphabetical Order sort($colors); echo "<p>"; foreach ($colors as $key => $value) { echo($key == 0) ? $value : ",".$value; } echo "</p>"; //Black, Blue, Brown, Green, Grey, Orange, Pink, Red, Yellow,Purple,White //Without Sorting $numbers = array(10, 32, 20, 1, 36, 12, 66, 72, 38, 18, 42, 90, 26, 73, 96, 53); echo "<p>"; foreach ($numbers as $key => $value) { echo($key == 0) ? $value: ",".$value; } echo "</p>"; //10,32,20,1,36,12,66,72,38,18,42,90,26,73,96,53 //After Sorting Array in Ascending Numerical Order sort($numbers); echo "<p>"; foreach ($numbers as $key => $value) { echo($key == 0) ? $value : ",$value"; } echo "</p>"; //1,10,12,18,20,26,32,36,38,42,53,66,72,73,90,96
2. Rsort rsort(array)
This function sorts an array in reverse order. Elements will be arranged in Descending order from highest to lowest when this function has completed.
Example Code:
//Without Sorting $colors = array(" White", " Black", " Grey", " Yellow", " Red", " Blue", " Green", " Brown", " Pink", " Orange", " Purple"); echo "<p>"; foreach ($colors as $key => $value) { echo($key == 0) ? $value : ",".$value; } echo "</p>"; //White, Black, Grey, Yellow, Red, Blue, Green, Brown, Pink, Orange,Purple //After Sorting Array in Descending Alphabetical Order rsort($colors); echo "<p>"; foreach ($colors as $key => $value) { echo($key == 0) ? $value : ",".$value; } echo "</p>"; //Yellow, White, Red, Purple, Pink, Orange, Grey, Green, Brown, Blue, Black //Without Sorting $numbers = array(10, 32, 20, 1, 36, 12, 66, 72, 38, 18, 42, 90, 26, 73, 96, 53); echo "<p>"; foreach ($numbers as $key => $value) { echo($key == 0) ? $value : ",".$value; } echo "</p>"; //10,32,20,1,36,12,66,72,38,18,42,90,26,73,96,53 //After Sorting Array in Descending Numerical Order rsort($numbers); echo "<p>"; foreach ($numbers as $key => $value) { echo($key == 0) ? $value : ",$value"; } echo "</p>"; //96,90,73,72,66,53,42,38,36,32,26,20,18,12,10,1
3. Asort asort(array)
This function sorts an array and maintain index association. Elements will be arranged in Ascending Order from lowest to highest with maintained index associated with each element of Array which Means After Sorting Key is fixed with value, when ever value is sorted key is moved with value.
Example Code:
//Without Sort $colors = array("White", " Black", " Grey", " Yellow", " Red", " Blue", " Green", " Brown", " Pink", " Orange", "Purple"); echo "<p>"; $i=0; foreach ($colors as $key => $value) { echo($i == 0) ? "$key => ".$value : ",$key => ".$value; $i++; } echo "</p>"; //0 => White,1 => Black,2 => Grey,3 => Yellow,4 => Red,5 => Blue,6 => Green,7 => Brown,8 => Pink,9 => Orange,10 => Purple //After Sorting Array in Ascending Alphabetical Order, According To Value and Maintain Index key Association asort($colors); echo "<p>"; $i=0; foreach ($colors as $key => $value) { echo($i == 0) ? "$key => ".$value : ",$key => ".$value; $i++; } echo "</p>"; //1 => Black,5 => Blue,7 => Brown,6 => Green,2 => Grey,9 => Orange,8 => Pink,4 => Red,3 => Yellow,10 => Purple,0 => White //Without Sort $numbers = array(10, 32, 20, 1, 36, 12, 66, 72, 38, 18, 42, 90, 26, 73, 96, 53); echo "<p>"; $i=0; foreach ($numbers as $key => $value) { echo($i == 0) ? "$key => ".$value : ",$key => ".$value; $i++; } echo "</p>"; //0 => 10,1 => 32,2 => 20,3 => 1,4 => 36,5 => 12,6 => 66,7 => 72,8 => 38,9 => 18,10 => 42,11 => 90,12 => 26,13 => 73,14 => 96,15 => 530 //After Sorting Array in Numerical Ascending Order , According To Value and Maintain Index key Association asort($numbers); echo "<p>"; $i = 0; foreach ($numbers as $key => $value) { echo($i == 0) ? "$key => ".$value : ",$key => ".$value; $i++; } echo "</p>"; //3 => 1,0 => 10,5 => 12,9 => 18,2 => 20,12 => 26,1 => 32,4 => 36,8 => 38,10 => 42,15 => 53,6 => 66,7 => 72,13 => 73,11 => 90,14 => 96
4. Arsort arsort(array)
This function sorts an array in reverse order and maintain index association. Elements will be arranged from highest to lowest with maintained index associated with each element of Array which Means After Sorting Key is fixed with value, when ever value is sorted key is moved with value.
Example Code:
//Without Sort $colors = array("White", " Black", " Grey", " Yellow", " Red", " Blue", " Green", " Brown", " Pink", " Orange", "Purple"); echo "<p>"; $i=0; foreach ($colors as $key => $value) { echo($i == 0) ? "$key => ".$value : ",$key => ".$value; $i++; } echo "</p>"; //0 => White,1 => Black,2 => Grey,3 => Yellow,4 => Red,5 => Blue,6 => Green,7 => Brown,8 => Pink,9 => Orange,10 => Purple //After Sorting Array in Descending Alphabetical Order, According To Value and Maintain Index key Association arsort($colors); echo "<p>"; $i=0; foreach ($colors as $key => $value) { echo($i == 0) ? "$key => ".$value : ",$key => ".$value; $i++; } echo "</p>"; //0 => White,10 => Purple,3 => Yellow,4 => Red,8 => Pink,9 => Orange,2 => Grey,6 => Green,7 => Brown,5 => Blue,1 => Black //Without Sort $numbers = array(10, 32, 20, 1, 36, 12, 66, 72, 38, 18, 42, 90, 26, 73, 96, 53); echo "<p>"; $i=0; foreach ($numbers as $key => $value) { echo($i == 0) ? "$key => ".$value : ",$key => ".$value; $i++; } echo "</p>"; //0 => 10,1 => 32,2 => 20,3 => 1,4 => 36,5 => 12,6 => 66,7 => 72,8 => 38,9 => 18,10 => 42,11 => 90,12 => 26,13 => 73,14 => 96,15 => 530 //After Sorting Array in Numerical Descending Order , According To Value and Maintain Index key Association arsort($numbers); echo "<p>"; $i = 0; foreach ($numbers as $key => $value) { echo($i == 0) ? "$key => ".$value : ",$key => ".$value; $i++; } echo "</p>"; //14 => 96,11 => 90,13 => 73,7 => 72,6 => 66,15 => 53,10 => 42,8 => 38,4 => 36,1 => 32,12 => 26,2 => 20,9 => 18,5 => 12,0 => 10,3 => 1
5. Ksort ksort()
This function sorts an array by key in ascending order . Elements will be arranged according to key order lowest to highest when this function has completed.
Example Code:
//Without Sort $colors = array(0 => "White",10 => "Purple",3 => "Yellow",4 => "Red",8 => "Pink",9 => "Orange",2 => "Grey",6 => "Green",7 => "Brown",5 => "Blue",1 => "Black"); echo "<p>"; $i=0; foreach ($colors as $key => $value) { echo($i == 0) ? "$key => ".$value : ",$key => ".$value; $i++; } echo "</p>"; //0 => White,10 => Purple,3 => Yellow,4 => Red,8 => Pink,9 => Orange,2 => Grey,6 => Green,7 => Brown,5 => Blue,1 => Black //After Sorting Array in Ascending Order, According To Index key Association ksort($colors); echo "<p>"; $i=0; foreach ($colors as $key => $value) { echo($i == 0) ? "$key => ".$value : ",$key => ".$value; $i++; } echo "</p>"; //0 => White,1 => Black,2 => Grey,3 => Yellow,4 => Red,5 => Blue,6 => Green,7 => Brown,8 => Pink,9 => Orange,10 => Purple //Without Sort $numbers = array(14 => 96,11 => 90,13 => 73,7 => 72,6 => 66,15 => 53,10 => 42,8 => 38,4 => 36,1 => 32,12 => 26,2 => 20,9 => 18,5 => 12,0 => 10,3 => 1); echo "<p>"; $i=0; foreach ($numbers as $key => $value) { echo($i == 0) ? "$key => ".$value : ",$key => ".$value; $i++; } echo "</p>"; //14 => 96,11 => 90,13 => 73,7 => 72,6 => 66,15 => 53,10 => 42,8 => 38,4 => 36,1 => 32,12 => 26,2 => 20,9 => 18,5 => 12,0 => 10,3 => 1 //After Sorting Array in Ascending Order , According To Index key Association ksort($numbers); echo "<p>"; $i = 0; foreach ($numbers as $key => $value) { echo($i == 0) ? "$key => ".$value : ",$key => ".$value; $i++; } echo "</p>"; //0 => 10,1 => 32,2 => 20,3 => 1,4 => 36,5 => 12,6 => 66,7 => 72,8 => 38,9 => 18,10 => 42,11 => 90,12 => 26,13 => 73,14 => 96,15 => 53
6. Krsort krsort()
This function sorts an array by key in reverse or descending order. Elements will be arranged according to key order highest to lowest when this function has completed.
Example Code:
//Without Sort $colors = array(0 => "White",10 => "Purple",3 => "Yellow",4 => "Red",8 => "Pink",9 => "Orange",2 => "Grey",6 => "Green",7 => "Brown",5 => "Blue",1 => "Black"); echo "<p>"; $i=0; foreach ($colors as $key => $value) { echo($i == 0) ? "$key => ".$value : ",$key => ".$value; $i++; } echo "</p>"; //0 => White,10 => Purple,3 => Yellow,4 => Red,8 => Pink,9 => Orange,2 => Grey,6 => Green,7 => Brown,5 => Blue,1 => Black //After Sorting Array in Descending Order, According To Index key Association krsort($colors); echo "<p>"; $i=0; foreach ($colors as $key => $value) { echo($i == 0) ? "$key => ".$value : ",$key => ".$value; $i++; } echo "</p>"; //10 => Purple,9 => Orange,8 => Pink,7 => Brown,6 => Green,5 => Blue,4 => Red,3 => Yellow,2 => Grey,1 => Black,0 => White //Without Sort $numbers = array(14 => 96,11 => 90,13 => 73,7 => 72,6 => 66,15 => 53,10 => 42,8 => 38,4 => 36,1 => 32,12 => 26,2 => 20,9 => 18,5 => 12,0 => 10,3 => 1); echo "<p>"; $i=0; foreach ($numbers as $key => $value) { echo($i == 0) ? "$key => ".$value : ",$key => ".$value; $i++; } echo "</p>"; //14 => 96,11 => 90,13 => 73,7 => 72,6 => 66,15 => 53,10 => 42,8 => 38,4 => 36,1 => 32,12 => 26,2 => 20,9 => 18,5 => 12,0 => 10,3 => 1 //After Sorting Array in Descending Order , According To Index key Association krsort($numbers); echo "<p>"; $i = 0; foreach ($numbers as $key => $value) { echo($i == 0) ? "$key => ".$value : ",$key => ".$value; $i++; } echo "</p>"; //15 => 53,14 => 96,13 => 73,12 => 26,11 => 90,10 => 42,9 => 18,8 => 38,7 => 72,6 => 66,5 => 12,4 => 36,3 => 1,2 => 20,1 => 32,0 => 10
Live Demo Sorting
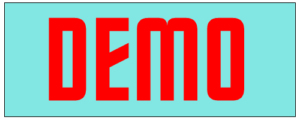