Why We Need Communication Between Two Arduino ?
Communication is Always need in Arduino When Working on Large projects where Single Arduino Not Handle Load Fully Functionality , We Having Long Distance or We Having Lots of Sensors Are Attach to Arduino and Arduino not handle all data process.Then we need Master And Slave Method by which data processing and data collection are divided into two boards.
List of Method to Communicate ?
- Serial Communication
- I2c
- Spi
Hardware Requirements
- Arduino Uno
- Arduino Mega Board
- Breadboard one or two
- Some Jumper wires
- Few Leds / Resistance also 100Ω.
- Push Buttons
Method Serial Communication
Example 1
In Example 1 Arduino Mega Having 4 Uart And Arduino Uno Having One Uart But We Need Two Uart in Arduino Uno So We need to Created Virtual Uart So See in Serial Port Moniter What data is Received.
The Serial Communication is Depended on Uart. As Many Uart Required To Send And Received Data Information Between Arduino Boards
Processing Algorithm
Circuit Diagram
Programming Code
Arduino Mega Code
int flag_led = 0; void setup() { Serial.begin(9600); Serial1.begin(9600); } void loop() { if (Serial.available() > 0) { while (Serial.available() > 0) { flag_led = Serial.read() - '0'; } } Serial1.print(flag_led); Serial.print(flag_led); Serial.println("-send-"); delay(1000); }
Arduino Uno Code
#define Ledpin 8 int recived = 0; #include <SoftwareSerial.h> //RX = digital pin 10, TX = digital pin 11 SoftwareSerial virtualPort(10, 11); void setup() { Serial.begin(9600); pinMode(Ledpin, OUTPUT); virtualPort.begin(9600); } void loop() { if(virtualPort.available()){ while (virtualPort.available() > 0) { recived = virtualPort.read()-'0'; } } Serial.print(recived); Serial.println("--recived--"); if (recived == 1) { digitalWrite(Ledpin, HIGH); } else { digitalWrite(Ledpin, LOW); } delay(1000); }
Result
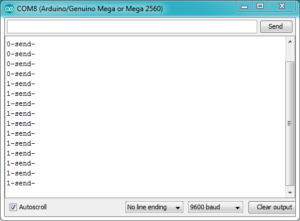
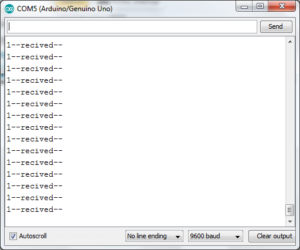
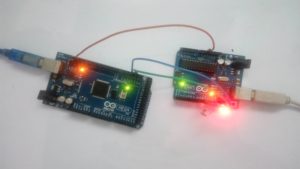
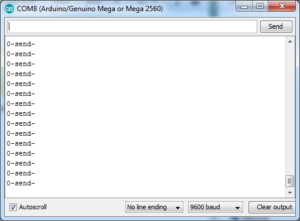
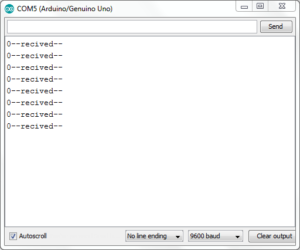
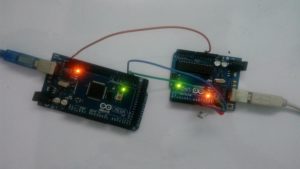
Example 2
In Example 2 Arduino Mega And Arduino Uno Not Use Serial Port Moniter so we use TX0 & RX0. During Upload Code Disconnect RX TX wires if You Connect Them And Try to Upload it is Impossible to upload Code so It better u dissconnect wires before Upload it.
Processing Algorithm
Circuit Diagram
Programming Code
Arduino Mega Code
int flag_led = 0; #define Buttonpin 7 void setup() { Serial.begin(9600); pinMode(Buttonpin, INPUT); } void loop() { if(digitalRead(Buttonpin)){ flag_led = 1; }else{ flag_led =0; } Serial.print(flag_led); delay(1000); }
Arduino Uno Code
#define Ledpin 8 int recived = 0; void setup() { Serial.begin(9600); pinMode(Ledpin, OUTPUT); } void loop() { if(Serial.available()){ while (Serial.available() > 0) { recived=0; recived = Serial.read()-'0'; } } if (recived == 1) { digitalWrite(Ledpin, HIGH); } else { digitalWrite(Ledpin, LOW); } delay(100); }
Result